Training, training, training!
1) Search for encapsulation in java... can you explain it in your own words?
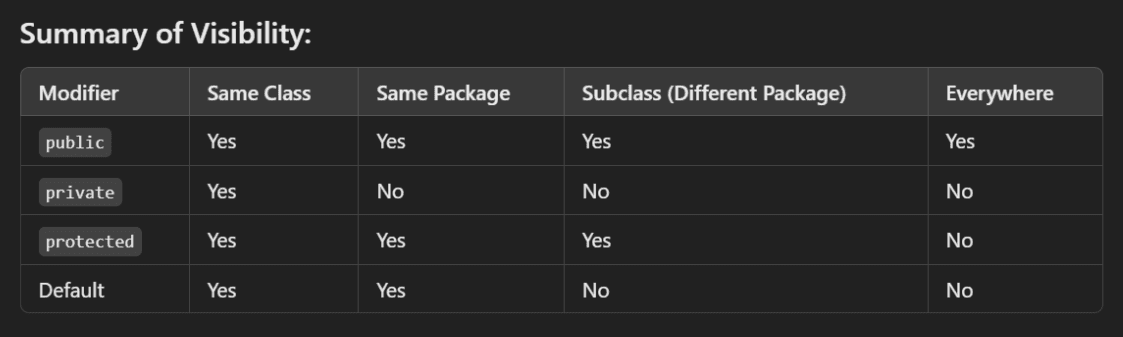
Prepare some examples to show in a project!
2) Collections!
Create a project and try to add and remove data from different collections. Can you identify the differences between Lists, Sets and Maps? Do you notice that these collections are interfaces? Why is an ArrayList an implementation of List?
3) Students and their grades!
Suppose we want to associate students and their grades from 0 to 20: Andre : 15 Barbara: 12 Daniel: 9 Eddie: 6 Jerry: 11 John: 20 Kurt: 10 William: 4 Robin: 18 Michael: 12 Create a collection that would keep this data.
4) We often need to change the content of the data...
Write a method that would remove a student receiving its name.
5) Removing really bad grades
Write a method that would remove every student that had less than 5.
6) Sort it!
Write a method to sort the collection either by grades or by the student's name.
7) Does it contain 'Andre'?
Write a method that would return if the collection has a student name in it, ignoring upper or lower case.
8) Print it!
Write a method that loops through this collection and prints both the student and their respective grade!
9) Count the occurrences!
Write a method that receives an integer and counts how many students had this grade!
10) Creating an HashSet!
Create an HashSet with the following Strings: - apple - grape - apple - onion - tomato - tomato What's the size of this Set?
11) Integer or int?
Create an HashSet with integers {1, 28, 19, 12, 13, 92}. Write a method that would sum all of the set entries. Why can't you use Set< int > but need Set< Integer > ? Investigate what is unboxing in java and prepare to explain it!
12) Abstract classes...
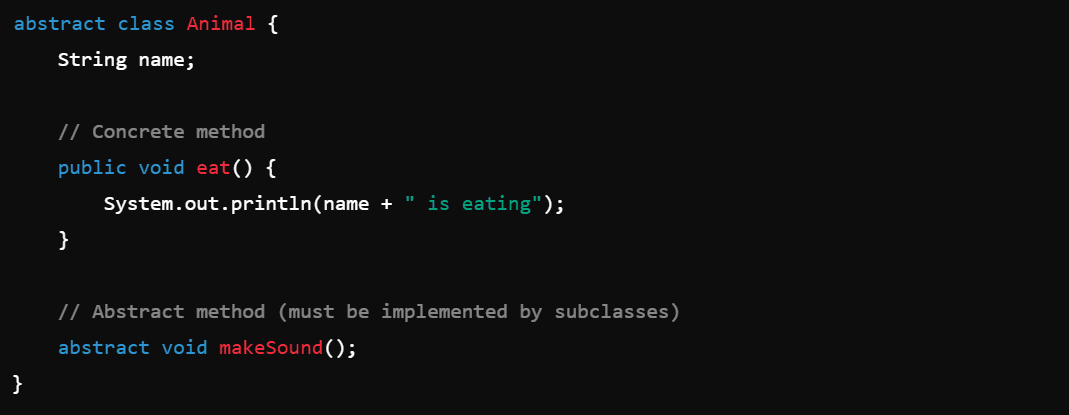
Can you explain this class?
13) Thinking about the snake game...
Consider the following classes: - Position - Snake - Food - Drawable - Movable - Collidable - Game - Board - Direction - Settings - Scoreboard - Element Which of these are classes (abstract or not), interfaces or enums? Can you draw their interactions, fields and dependencies? hint: you can use tldraw.com to create a diagram!